Common Mistakes to Avoid When Assigning Variables in Python
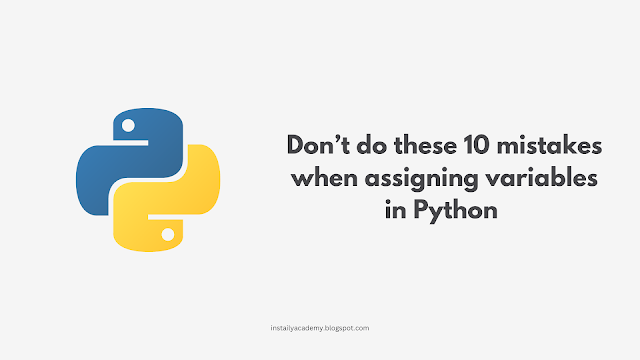
In the world of Python programming, variable assignment is a fundamental concept. It allows you to store and manipulate data, making it a crucial skill for both beginners and experienced developers. However, like any aspect of coding, there are common mistakes that can trip you up along the way. In this article, we'll explore some of the most frequent pitfalls to avoid when assigning variables in Python, ensuring that your code remains clean, efficient, and error-free.
Understanding Variable Assignment in Python
Before we delve into common mistakes, let's clarify what variable assignment in Python entails. When you assign a variable, you're essentially creating a name for a piece of data, whether it's a number, string, list, or more complex object. Python allows for dynamic typing, meaning you don't need to declare the variable's type explicitly.Mistake #1: Overwriting Variables
One of the most frequent errors is overwriting variables unintentionally. If you assign a new value to an existing variable, it erases the previous value. Always be cautious when reusing variable names to avoid unexpected behavior.Mistake #2: Using Reserved Keywords
Python has reserved keywords like "if," "else," and "while" that cannot be used as variable names. Attempting to do so will result in syntax errors.Mistake #3: Uninitialized Variables
Trying to use a variable before assigning it a value leads to a "NameError." Ensure every variable is initialized before use.Mistake #4: Mixing Variable Types
Python allows dynamic typing, but it's essential to be aware of the variable type you're working with. Mixing types can lead to unexpected results.Mistake #5: Ignoring Scope
Variables have scope, meaning they exist within certain parts of your code. Not understanding scope can result in errors or unintended side effects.Mistake #6: Not Using Descriptive Names
Clear and descriptive variable names enhance code readability. Avoid cryptic or overly short names that make your code hard to understand.Mistake #7: Not Updating Variables Correctly
When updating variables, ensure you perform the correct operations. For example, use += for incrementing, not =.Mistake #8: Failing to Check for None
If a variable can be None, always check for it before using the variable to avoid "NoneType" errors.Mistake #9: Not Using Tuple Unpacking
Tuple unpacking can simplify variable assignment, especially when dealing with multiple values.Mistake #10: Neglecting Type Casting
Explicitly convert variable types when necessary. Neglecting this can lead to unexpected results in operations.Best Practices for Variable Assignment
To avoid these common mistakes, consider the following best practices:- Use descriptive variable names.
- Initialize variables before use.
- Be mindful of variable scope.
- Check for None before using variables.
- Explicitly cast variable types when needed.
Comments
Post a Comment